Mastering Arrow Functions in JavaScript: Syntax, Behavior, and Best Practices
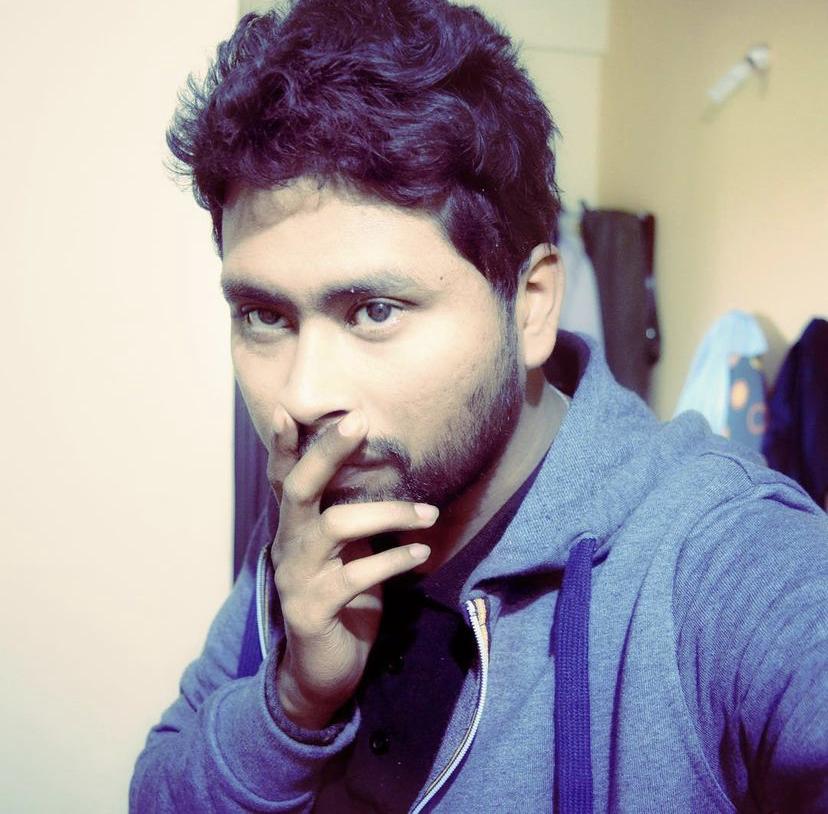
What Are Arrow Functions?
Arrow functions (introduced in ES6) are like the "express lane" of JavaScript functions. They provide:
-
Shorter syntax (like a condensed function declaration)
-
No binding of this (they inherit context like a child inherits eye color)
-
Implicit returns for single expressions (automatic value delivery)
Traditional Function vs. Arrow Function Analogy
Imagine ordering coffee:
Regular function: "I'd like a coffee, please wait while I brew it, then return it to you."
Arrow function: "Coffee, black." (direct and concise)
Function Types in JavaScript: Quick Refresher
1. Function Declarations (Hoisted) Function declarations (Hoisted) are a way to define functions in JavaScript that are hoisted, meaning they can be called before they are defined in the code. This happens because the JavaScript engine moves the function declarations to the top of their containing scope during the compilation phase.
1function add(x, y) {
2 return x + y;
3}
4
2. Function Expressions (Not Hoisted) Function expressions are another way to define functions in JavaScript. Unlike function declarations, function expressions are not hoisted, meaning they cannot be called before they are defined in the code.
1const subtract = function (x, y) {
2 return x - y;
3};
4
3. Generator Functions (Yield Multiple Values) These functions can yield multiple values, allowing you to pause and resume their execution. They are defined using the function*
syntax and the yield
keyword.
1function* countTo3() {
2 yield 1;
3 yield 2;
4 yield 3;
5}
6
4. Arrow Functions (Concise Syntax) Arrow functions are a more concise way to write functions in JavaScript. They are defined using the =>
syntax and have implicit returns for single expressions.
1const multiply = (x, y) => x * y;
2
Arrow Function Syntax Deep Dive
This section provides an in-depth look at the syntax of arrow functions in JavaScript, highlighting their concise and direct nature compared to traditional functions.
Basic Structure of Arrow Functions
The basic structure of an arrow function consists of the following elements: parameters, the arrow (=>), and the function body.
Traditional function
1function add(x, y) {
2 return x + y;
3}
4
Converted to arrow function
1const add = (x, y) => x + y;
2
Try in editor:
Like a vending machine vs. a waiter:
Arrow functions can have an implicit return, meaning they automatically return the result of the expression without needing the return keyword. This is similar to a vending machine that gives you what you want without any extra steps.
On the other hand, if you use curly braces , you must explicitly use return to get a result, like a waiter who needs to be told to bring your order.
Implicit return (vending machine: input → immediate output)
1const isEven = (num) => num % 2 === 0;
2
Explicit return (waiter: multiple steps before delivery)
1const isEvenVerbose = (num) => {
2 console.log(`Checking if ${num} is even...`);
3 return num % 2 === 0;
4};
5console.log(isEven(4)); // true
6console.log(isEvenVerbose(4)); // Checking if 4 is even... true
7
Traditional Function Pain Point In traditional functions, the value of this can be tricky because it depends on how the function is called. This often leads to confusion and errors, especially when functions are used as callbacks or event handlers.
Arrow functions solve this problem by not having their own this context. Instead, they inherit this from the surrounding code, making them easier to use in many situations.
1function BrokenTimer() {
2 this.seconds = 0;
3 setInterval(function () {
4 // `this` refers to window, not Timer instance!
5 this.seconds++;
6 console.log("Broken:", this.seconds); // NaN
7 }, 1000);
8}
9// new BrokenTimer(); // Uncomment to see broken behavior
10
Arrow Function Fix
1function WorkingTimer() {
2 this.seconds = 0;
3 setInterval(() => {
4 this.seconds++;
5 console.log("Working:", this.seconds); // Correctly increments
6 }, 1000);
7}
8// new WorkingTimer(); // Uncomment to see correct behavior
9
Analogy: Arrow functions are like security cameras - they capture their surrounding context (this) when installed and keep that perspective forever.
When NOT to Use Arrow Functions
Arrow functions are great for many situations, but there are times when they are not suitable. Avoid using arrow functions when you need a function to have its own this context, such as in methods of an object, constructors, or when you need to use the arguments object.
- Object Methods Arrow functions should not be used as methods in objects. This is because arrow functions do not have their own this context; they inherit this from the surrounding scope. When used in an object method, this behavior can lead to unexpected results, as the this keyword will not refer to the object itself, but to the outer scope where the method is defined. This can cause errors when trying to access or modify the object's properties
1const badAccount = {
2 balance: 1000,
3 withdraw() {
4 // Correctly refers to `this` of the object
5 this.balance -= 100; // Will work now!
6 },
7};
8badAccount.withdraw();
9console.log("Fixed balance:", badAccount.balance); // 900
10
- Event Handlers Arrow functions should not be used for event handlers because they do not have their own this context. Instead, they inherit this from the surrounding scope. In event handlers, this usually refers to the element that triggered the event. Using an arrow function can cause this to point to the wrong context, leading to unexpected behavior and making it difficult to interact with the event-triggering element.
- Prototype Methods Arrow functions should not be used in prototype methods because they do not have their own this context. Instead, they inherit this from the surrounding scope, which can lead to errors when trying to access or modify properties of the object. In prototype methods, this should refer to the instance of the object, but using an arrow function can cause this to point to the wrong context, resulting in unexpected behavior.
1function Person(name) { this.name = name; }
2
3// WRONG:
4Person.prototype.greet = () => console.log('Hi, I'm', this.name); // undefined
5
6// RIGHT:
7Person.prototype.greet = function() { console.log('Hi, I'm', this.name); };
8const alice = new Person('Alice');
9alice.greet();
10
Advanced Edge Cases & Solutions
- Returning Object Literals
In JavaScript, when using arrow functions, the syntax can sometimes lead to unexpected behavior, especially when returning object literals.
In the broken example:
1const makeUser = () => { name: 'Alice', age: 30 };
2
The curly braces {}
are interpreted as the start of a function body, not an object literal. As a result, the function does not return the object as intended.
To fix this, you can wrap the object literal in parentheses:
1const makeUser = () => ({ name: "Alice", age: 30 });
2
By using parentheses, you explicitly indicate that the curly braces are for an object literal, not a function body. This ensures that the function returns the object { name: 'Alice', age: 30 }
as expected. The console.log(makeUser());
will then output the object to the console.
- Line Break Sensitivity
In JavaScript, arrow functions have specific syntax rules regarding line breaks.
In the syntax error example:
1const add = (a, b)
2 => a + b;
3
The line break between the parameters (a, b)
and the arrow =>
causes a syntax error. JavaScript expects the arrow =>
to immediately follow the parameter list without a line break.
In the valid example:
1const safeAdd = (a, b) => a + b;
2
The arrow =>
directly follows the parameter list on the same line, which is correct. The expression a + b
can be on the next line because it is part of the function body. This syntax is valid and will work as expected.
- Arguments Object Limitation
Arrow functions do not have their ownarguments
object, unlike traditional functions. This can lead to errors when trying to access the arguments passed to an arrow function. Moreover, arrow functions lack thearguments
object, which is a feature of traditional functions. Thearguments
object is an array-like object that contains all the arguments passed to a function.
In JavaScript, traditional functions have access to the arguments
object, which is an array-like object containing all the arguments passed to the function. This allows you to log or manipulate the arguments easily, as shown in the traditional function example:
1const traditional = function (a, b) {
2 console.log("Arguments:", arguments); // Works
3};
4
However, arrow functions do not have their own arguments
object. Instead, they inherit arguments
from the enclosing scope, which can lead to a ReferenceError
if there is no arguments
object in the surrounding context. This is why the arrow function example results in an error:
1const arrow = (a, b) => {
2 console.log("Arguments:", arguments); // ReferenceError
3};
4
To handle multiple arguments in arrow functions, you can use rest parameters (...args
) instead.
Here's an example of using rest parameters in an arrow function to handle multiple arguments:
1const sum = (...args) => {
2 return args.reduce((total, current) => total + current, 0);
3};
4
5console.log(sum(1, 2, 3, 4)); // Outputs: 10
6
In this example, the sum
arrow function uses the rest parameter syntax ...args
to collect all arguments into an array called args
. The reduce
method is then used to calculate the sum of all the numbers in the array.
FAQ: Common Arrow Function Questions
No - they lack [[Construct]]
capability:
1const Car = () => {};
2const myCar = new Car(); // TypeError: Car is not a constructor
3
No - use rest parameters instead:
1const sum = (...args) => args.reduce((total, num) => total + num, 0);
2
No - syntax forbids yield in arrow functions.
They're like tattoos - permanently bound to their creation context:
1const printThis = () => console.log(this);
2const boundPrint = printThis.bind({ custom: "object" });
3boundPrint(); // Still shows original `this`
4
They behave like other variables (not hoisted):
1console.log(square(5)); // Error
2const square = (x) => x * x;
3
Not for methods needing this:
1class BadClass {
2 value = 10;
3 print = () => console.log(this.value); // Works but memory inefficient
4}
5
Same as regular functions:
1const fetchData = async () => {
2 const res = await fetch("api.url");
3 return res.json();
4};
5
Negligible difference in modern engines - prioritize readability.
Yes, but name them first:
1const factorial = (n) => (n <= 1 ? 1 : n * factorial(n - 1));
2
Name them for better stack traces:
1const bad = (x) => x * 2; // Shows as "anonymous"
2const good = function goodName(x) {
3 return x * 2;
4};
5
Conclusion & Next Steps
Key Takeaways:
- Use arrows for concise callbacks and lexical this
- Avoid them for object methods and constructors
- Master implicit returns and parameter handling